일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- git
- 솔로드릴
- development
- 개발자
- 영화
- graphQL
- 웹개발
- 주짓수
- 영화감상
- 드릴
- Redux
- 자바스크립트
- 엄티로드
- Node
- 영화리뷰
- 클로즈가드
- web
- 노드
- 파이썬
- 주짓떼로
- REACT
- nodejs
- 하프가드
- 디자인패턴
- 개발
- 주짓떼라
- JavaScript
- Express
- 프로그래밍
- 리액트
- Today
- Total
As i wish
[Design pattern] Command pattern (커멘드패턴) 본문
오랜만에 포스팅인데요
오늘은 커멘드 패턴에 대하여 알아 보겠습니다.
일단 커멘드 패턴이란...
요청을 객체의 형태로 캡슐화하여 사용자가 보낸 요청을 나중에 이용할 수 있도록 매서드 이름, 매개변수 등 요청에 필요한 정보를 저장 또는 로깅, 취소할 수 있게 하는 패턴이다.
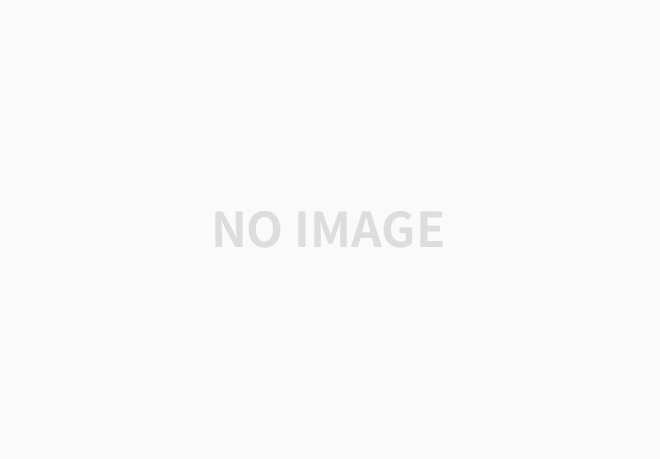
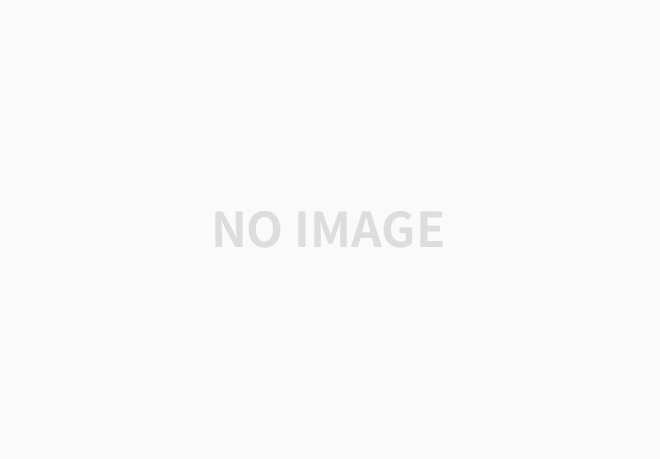
쉽게 설명하면 가게에서 주문을 하는 경우라고 설명 할 수 있겠는데요.
손님이 주문을 하면, 웨이터가 그 주문을 받고 주문에 따라 주방장에게 요청을 하면, 주방장이 주문에 따라서 각 요리를 만드는것으로 쉽게 비유해 볼 수 있겠네요.
여기서
손님은 client,
웨이터는 invoker,
주문서가 command,
손님이 웨이터에게 주문서를 주는 행위를 setCommand(),
웨이터가 주방장에게 주문서를 주는 행위를 execute(),
주방장이 receiver라고 이해하면 쉬울듯 하네요.
그럼 더욱 쉽게 코드로 확인해 보겠습니다.
역시나 저는 언제나 파이썬입니다.
코드의 내용은 책에서 설명한것 처럼 리모콘을 예로 들겠습니다.
리모콘에는 여러가지 버튼이 있는데요
불을 키는 버튼, 끄는 버튼,
tv를 키는 버튼, 끄는 버튼,
오디오를 키는 버튼, 끄는 버튼
이것을 앞서 설명한 주문서에 비교해 본다면
손님은 사용자 또는 RemoveLoader (Client),
웨이터는 RemoteControl (invoker),
주문서가 Buttons (command),
손님이 웨이터에게 주문서를 주는 행위가 버튼을 리모콘에 등록하는 행위 (setCommand()),
웨이터가 주방장에게 주문서를 주는 행위가 버튼을 누르는 행위 (execute()),
주방장이 버튼에 따라 발생하는 것들 (전등, tv, 오디오)(receiver)라고 대입하면 될듯 합니다.
# -*- coding: utf-8 -*-
# Command Pattern
import abc
## Invoker
class RemoteControl:
def __init__(self):
self.onCommand = []
self.offCommand = []
def setCommand(self, onCommand, offCommand):
self.onCommand.append(onCommand)
self.offCommand.append(offCommand)
def onButtonWasPushed(self, slot):
self.onCommand[slot].execute()
def offButtonWasPushed(self, slot):
self.offCommand[slot].execute()
## Receiver
class LightObj:
def on(self):
print ("Light ON")
def off(self):
print ("Light OFF")
class TvObj:
def on(self):
print ("TV ON")
def off(self):
print ("TV OFF")
class AudioObj:
def on(self):
print ("Audio ON")
def off(self):
print ("Audio OFF")
## Command
class Command:
__metaclass__ = abc.ABCMeta
@abc.abstractmethod
def execute(self):
pass
class LightOnCommand(Command):
def __init__(self, lightObject):
self.light = lightObject
def execute(self):
self.light.on()
class LightOffCommand(Command):
def __init__(self, lightObject):
self.light = lightObject
def execute(self):
self.light.off()
class TVOnCommand(Command):
def __init__(self, tvObject):
self.tv = tvObject
def execute(self):
self.tv.on()
class TVOffCommand(Command):
def __init__(self, tvObject):
self.tv = tvObject
def execute(self):
self.tv.off()
class AudioOnCommand(Command):
def __init__(self, audioObject):
self.audio = audioObject
def execute(self):
self.audio.on()
class AudioOffCommand(Command):
def __init__(self, audioObject):
self.audio = audioObject
def execute(self):
self.audio.off()
# Invoker
remoteContoller = RemoteControl()
# Reciever
lightObj = LightObj()
tvObj = TvObj()
audioObj = AudioObj()
# Command
lightOnButton = LightOnCommand(lightObj)
lightOffButton = LightOffCommand(lightObj)
tvOnButton = TVOnCommand(tvObj)
tvOffButton = TVOffCommand(tvObj)
audioOnButton= AudioOnCommand(audioObj)
audioOffButton = AudioOffCommand(audioObj)
# SetCommand
remoteContoller.setCommand(lightOnButton, lightOffButton)
remoteContoller.setCommand(tvOnButton, tvOffButton)
remoteContoller.setCommand(audioOnButton, audioOffButton)
# Execute
remoteContoller.onButtonWasPushed(0)
remoteContoller.onButtonWasPushed(1)
remoteContoller.onButtonWasPushed(2)
remoteContoller.offButtonWasPushed(0)
remoteContoller.offButtonWasPushed(1)
remoteContoller.offButtonWasPushed(2)
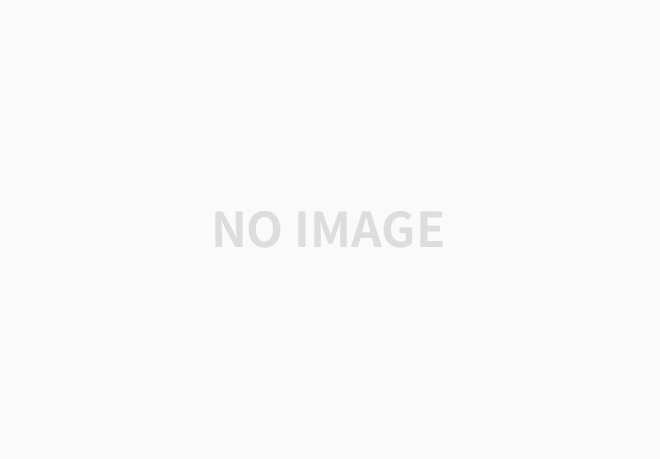
끝!!
'Design Pattern' 카테고리의 다른 글
[Design pattern] Facade pattern (퍼사드패턴) (0) | 2019.04.22 |
---|---|
[Design pattern] Adapter pattern (어댑터패턴) (0) | 2019.04.22 |
[Design pattern] Singleton pattern (싱글톤 패턴) (0) | 2019.03.10 |
[Design pattern] Abstact Factory pattern (추상 팩토리 패턴) (0) | 2019.02.17 |
[Design pattern] Factory method pattern (팩토리 메소드 패턴) (0) | 2019.02.10 |